Cross-chain Use-Cases: Deposit From Anywhere
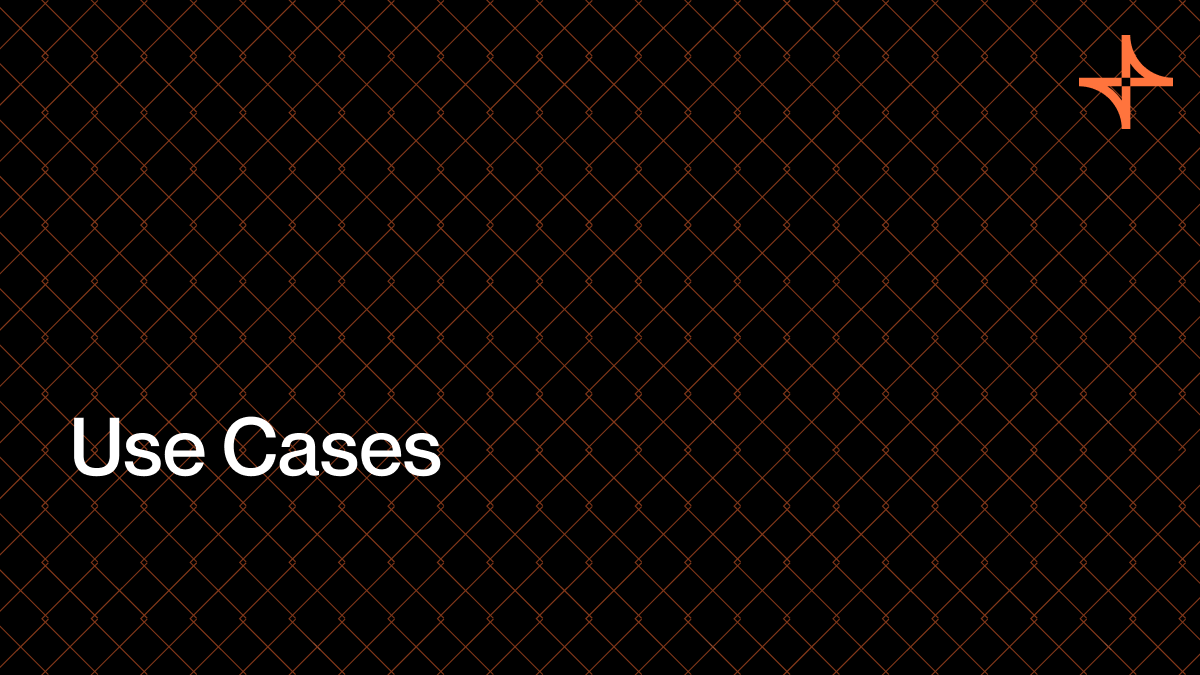
Sygma is designed to make adding cross-chain functionality to your application a low-effort task. In this sense, possibilities include bridging directly from a wallet or portfolio manager, integrating directly into any EVM or Substrate-based dApp, marketing NFTs seamlessly on any chain, or being able to vote on DAO governance proposals regardless of the chain.
This is just the tip of the iceberg, and in this article, we’ll focus on one of these in particular.
Deposit from anywhere
As a developer, your primary goal is to build value and provide a good user experience. If, for example, you’re building a DEX on Substrate, like HydraDX or Polkaswap, you could significantly enhance the user experience by enabling users to deposit assets into your dApp from any blockchain, especially EVM-based ones.
Let's say you're a developer named Blocky Balboa, and you want to add functionality that allows users to deposit tokens to your Substrate-based dApp from any chain (e.g., EVM-based chains) in just one click. Sygma makes this possible.
Below is a high-level overview of what would need to happen on the backend for you to integrate the Sygma SDK into your dApp:
Install the Sygma SDK
Written in TypeScript and available in a Node.js and browser environment, the SDK can be installed using npm or yarn.
To install using npm:
npm install @buildwithsygma/sygma-sdk-core
To install using yarn:
yarn add @buildwithsygma/sygma-sdk-core
Create a transfer object
Depending on whether the source network of the tokens is an EVM-based or a Substrate-based chain, Blocky will create an instance of either the EvmAssetTransfer or SubstrateAssetTransfer object, respectively.
From an EVM-based chain
const assetTransfer = new EvmAssetTransfer();
const provider = new JsonRpcProvider("https://URL-TO-YOUR-RPC")
await assetTransfer.init(
Provider,
Environment.TESTNET
);
From a Substrate-based chain
const assetTransfer = new SubstrateAssetTransfer();
const wsProvider = new WsProvider("wss://URL-TO-YOUR-SUBSTRATE-INSTANCE");
// Create an instance of the PolkadotJS ApiPromise
const api = await ApiPromise.create({ provider: wsProvider });
await assetTransfer.init(
Api,
Environment.TESTNET
);
Determine the transfer fee
The next step is to determine the fee for the transfer. This step can be done by utilizing the getFee() method on the asset transfer object. Blocky will need to know the destination ChainID and the ResourceID configured on the bridge.
For an EVM-based chain:
const wallet = new Wallet(
"YOUR PRIVATE KEY",
provider
);
const transfer = assetTransfer.createFungibleTransfer(
await wallet.getAddress(),
DESTINATION_CHAINID,
DESTINATION_ADDRESS,
RESOURCE_ID,
AMOUNT
)
const fee = await assetTransfer.getFee(transfer);
For a Substrate-based chain:
const keyring = new Keyring({ type: "sr25519" });
await cryptoWaitReady();
const account = keyring.addFromUri(MNEMONIC);
const transfer = assetTransfer.createFungibleTransfer(
account.address,
DESTINATION_CHAINID,
DESTINATION_ADDRESS,
RESOURCE_ID,
AMOUNT
)
const fee = await assetTransfer.getFee(transfer);
Check and send approvals
If the source network is an EVM-based chain, Blocky may need to check if they need approvals for the transfer.
// Build approvals given the `transfer` and `fee` parameters
const approvals = await assetTransfer.buildApprovals(transfer, fee);
// Check if approvals are needed
if (approvals.length) {
for (const approval of approvals) {
const approvalTx = await wallet.sendTransaction(approval as providers.TransactionRequest);
console.log(`Approval TX Hash: ${approvalTx.hash}`)
}
}
Prepare and send the transfer transaction
Blocky must then prepare, sign, and send the transfer transaction to the source network node.
For an EVM-based chain:
// Build the transfer transaction
const transferTransaction = await assetTransfer.buildTransferTransaction(
transfer,
fee,
);
// Send the transaction using the wallet
const transferTxResponse = await wallet.sendTransaction(
transferTx as providers.TransactionRequest,
);
For a Substrate-based chain:
// Build the transfer transaction
const transferTransaction = await assetTransfer.buildTransferTransaction(
transfer,
fee
);
// Sign and broadcast the transfer transaction
const unsub = await transferTransaction.signAndSend(account, ({ status }) => {
console.log(`Current status is ${status.toString()}`);
if (status.isInBlock) {
console.log(
`Transaction included at blockHash ${status.asInBlock.toString()}`
);
} else if (status.isFinalized) {
console.log(
`Transaction finalized at blockHash ${status.asFinalized.toString()}`
);
unsub();
}
});
6. Confirm the Transaction
Blocky should then confirm the transaction by checking the transaction hash in a block explorer.
// Print the transaction hash
console.log(`Transfer sent with hash: ${transferTxResponse.hash}`)
The above steps provide a simple, high-level overview of how a developer might be able to integrate Sygma SDK into their dApp.
While it will be necessary to tinker further and dive into the Sygma repos to have a fully fleshed-out product, hopefully, this guide gives a sense of the potential. Blocky can also utilize the Sygma widget, a soon-to-be-released, fully customizable ‘one-stop-shop’ widget that makes integrating bridging and swaps easy.
Please refer to the full Sygma SDK documentation for a deeper understanding and for handling more complex scenarios.
Community👥
Sygma connects you with the chains you want, making it possible to compose applications that work across EVM, Substrate, and Cosmos.
The strength of our project lies in our builder community. We're always looking for contributors, and Sygma’s modular architecture is designed to foster contributions and extensions.
Check out our documentation or GitHub to get started.
Have a question? Hop into our Discord 👋